Python Regex with Paramiko
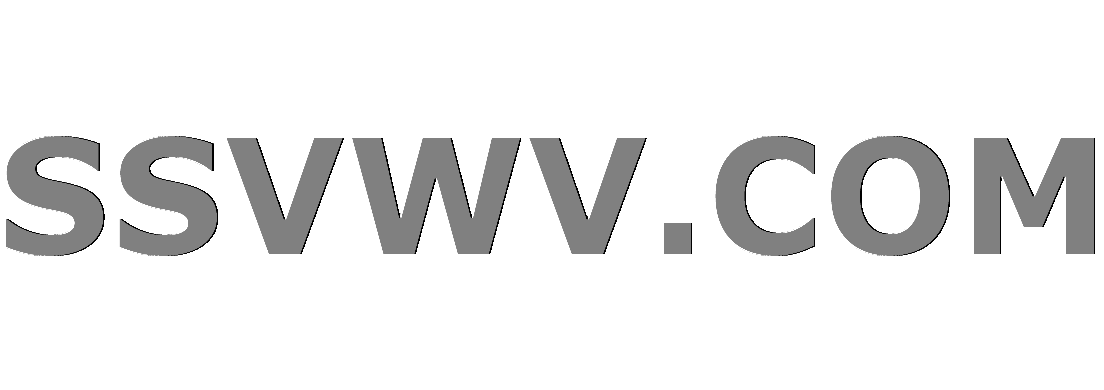
Multi tool use
Python Regex with Paramiko
I am using Python Paramiko module to sftp into one of my servers. I did a list_dir()
to get all of the files in the folder. Out of the folder I'd like to use regex to find the matching pattern and then printout the entire string.
list_dir()
List_dir will list a list of the XML files with this format
LOG_MMDDYYYY_HHMM.XML
LOG_07202018_2018 --> this is for the date 07/20/2018 at the time 20:18
Id like to use regex to file all the XML files for that particular date and store them to a list or a variable. I can then pass this variable to Paramiko to get the file.
for log in file_list:
regex_pattern = 'POSLog_' + date + '*'
if (re.search(regex_pattern, log) != None):
matchObject = re.findall(regex_pattern, log)
print(matchObject)
the code above just prints: ['Log_07202018']
I want it to store the entire string Log_07202018_20:18.XML
to a variable.
['Log_07202018']
Log_07202018_20:18.XML
How would I go about doing this?
Thank you
2 Answers
2
If you are looking for a fixed string, don't use regex.
search_str = 'POSLog_' + date
for line in file_list:
if search_str in line:
print(line)
Alternatively, a list comprehension can make list of matching lines in one go:
log_lines = [line for line in file_list if search_str in line]
for line in log_lines:
print(line)
If you must use regex, there are a few things to change:
*
.*
^
^
MULTILINE
There are several ways of getting all matches. One could do "for each line, if there is a match, print line", same as above. Here I'm using .finditer()
and a search over the whole input block (i.e. not split into lines).
.finditer()
log_pattern = '^POSLog_' + re.escape(date) + '.*'
for match in re.finditer(log_pattern, whole_file, re.MULTILINE):
print(match.string)
if search_str in line
You are absolutely right, answer modified.
– Tomalak
Aug 20 at 4:56
the string is fixed in the beginning part so it will always show POSLOG_MMDDYY_ but theHHMM at the end will change. Will for line in file_list: still print out? or does it have to be an exact match, no more no less?
– user3010500
Aug 20 at 6:08
You can answer this question by looking at the code.
– Tomalak
Aug 20 at 6:13
Keep getting this error. for match in re.finditer(log_pattern, file_list, re.MULTILINE): File "C:UsersKXM4328AppDataLocalProgramsPythonPython36libre.py", line 229, in finditer return _compile(pattern, flags).finditer(string) TypeError: expected string or bytes-like object
– user3010500
Aug 21 at 4:33
Because you only print the matched part, just do print(log)
instead and it'll print the whole filename.
print(log)
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Definetely, can even simply use
if search_str in line
, supposed to be faster.– Bernhard
Aug 20 at 4:55