How to join two arrays into one two-dimensional array?
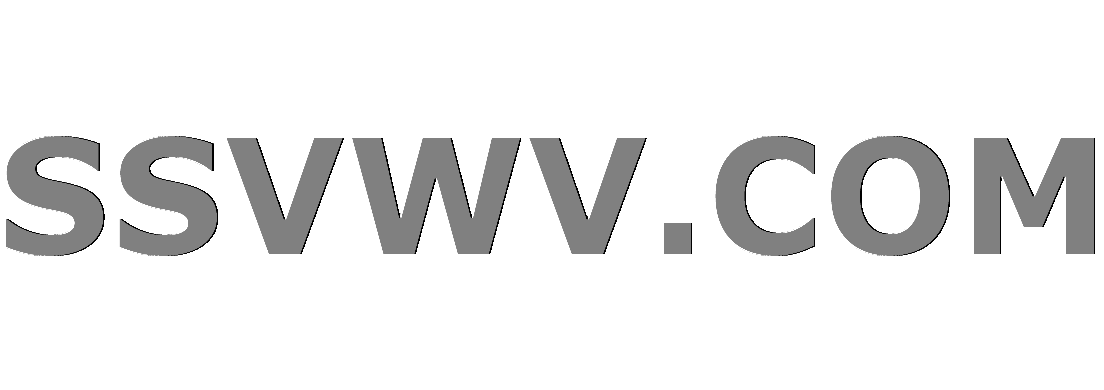
Multi tool use
How to join two arrays into one two-dimensional array?
I have two arrays. How can I join them into one multidimensional array?
The first array is:
var arrayA = ['Jhon, kend, 12, 62626262662',
'Lisa, Ann, 43, 672536452',
'Sophie, Lynn, 23, 636366363'];
My other array has the values:
var arrayB = ['Jhon', 'Lisa', 'Sophie'];
How could I get an array with this format??
var jarray = [['Jhon', ['Jhon, kend, 12, 62626262662']],
['Lisa', ['Lisa, Ann, 43, 672536452']],
['Sohphie', ['Sophie, Lynn, 23, 636366363']]]
Thanks for this question ... Helped
– Rush.2707
Aug 22 '16 at 5:26
4 Answers
4
var jarray = ;
for (var i=0; i<arrayA.length && i<arrayB.length; i++)
jarray[i] = [arrayB[i], [arrayA[i]]];
However, I wouldn't call that "multidimensional array" - that usually refers to arrays that include items of the same type. Also I'm not sure why you want the second part of your arrays be an one-element array.
Though you'd want to make sure the arrays are of equal length (or use the length of the smaller one).
– iamnotmaynard
Feb 11 '13 at 21:23
@iamnotmaynard: I already use the length of the smaller one. Of course, a
for (var i=0, l=Math.min(arrayA.length, arrayB.length); i<l; i++)
might have been more elegant.– Bergi
Feb 11 '13 at 21:25
for (var i=0, l=Math.min(arrayA.length, arrayB.length); i<l; i++)
Ah, I see I had interchanged them. Fixed now.
– Bergi
Feb 11 '13 at 21:28
@FelixKling: You think the OP actually had more than 3 strings in his
arrayA
? Yes, then you would need to adapt my solution a bit.– Bergi
Feb 12 '13 at 13:44
arrayA
Oh damn... I'm so sorry, I did not notice that those are three strings. I thought the array contains 12 elements! So sorry :-/ Will delete all my comments.
– Felix Kling
Feb 12 '13 at 14:00
You can use Underscore.js http://underscorejs.org/#find
Looks through each value in the list, returning the first one that passes a truth test (iterator). The function returns as soon as it finds an acceptable element, and doesn't traverse the entire list.
var even = _.find([1, 2, 3, 4, 5, 6], function(num) return num % 2 == 0; );
=> 2
Then, you can make the same with the array B elements and by code, make a join.
Hmm, really no. Because, he need find elements in the arrays A and B. And if he make a for (var i=0; i<arrayB.length;i++) he can know the names of elements in this array. with underscore, he can find and asociate this elements
– MrMins
Feb 11 '13 at 21:22
This is what I did to get what you were asking:
var jarray = ;
for (var i = 0; i < arrayB.length; i++)
jarray[i] = ;
jarray[i].push(arrayB[i]);
var valuesList = ,
comparator = new RegExp(arrayB[i]);
for (var e = 0; e < arrayA.length; e++)
if (comparator.test(arrayA[e]))
valuesList.push(arrayA[e]);
jarray[i].push(valuesList);
Here is a map version
var arrayA = ['Jhon, kend, 12, 62626262662',
'Lisa, Ann, 43, 672536452',
'Sophie, Lynn, 23, 636366363'];
var arrayB = ['Jhon', 'Lisa', 'Sophie'];
/* expected output
var jarray = [['Jhon', ['Jhon, kend, 12, 62626262662']],
['Lisa', ['Lisa, Ann, 43, 672536452']],
['Sohphie', ['Sophie, Lynn, 23, 636366363']]] */
var jarray = arrayB.map(function(item,i)
return [item,[arrayA[i]]];
);
console.log(jarray);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
This is called "zipping", here's the javascript implementation.
– georg
Feb 11 '13 at 21:23