What is the “-->” operator in C++?
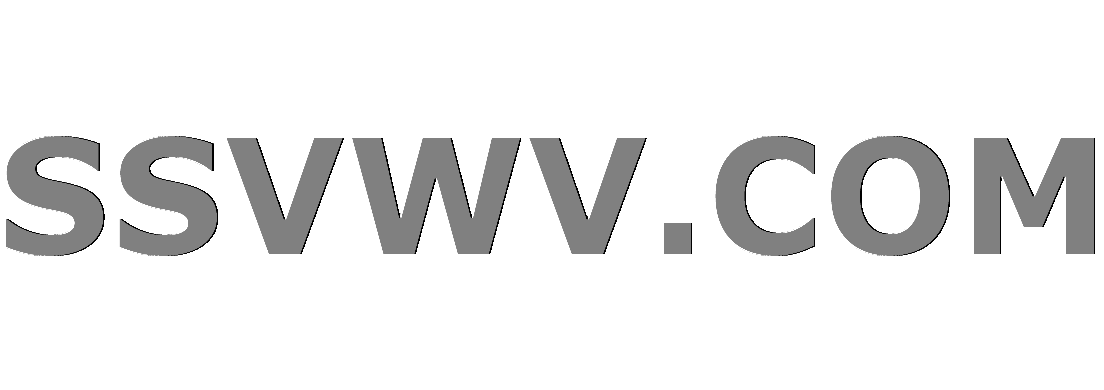
Multi tool use
What is the “-->” operator in C++?
After reading Hidden Features and Dark Corners of C++/STL on comp.lang.c++.moderated
, I was completely surprised that the following snippet compiled and worked in both Visual Studio 2008 and G++ 4.4.
comp.lang.c++.moderated
Here's the code:
#include <stdio.h>
int main()
int x = 10;
while (x --> 0) // x goes to 0
printf("%d ", x);
I'd assume this is C, since it works in GCC as well. Where is this defined in the standard, and where has it come from?
++
--
This "goes to" operator can be reverted ( 0 <-- x ). And also there is a "runs to" operator ( 0 <---- x ). Geez, the funniest thing I've ever heard of c++ syntax =) +1 for the question.
– SadSido
Oct 29 '09 at 7:27
Funnily enough, although the interpretation is very wrong, it does describe what the code does correctly. :)
– Noldorin
Nov 11 '09 at 13:51
Imagine the new syntax possibilities:
#define upto ++<
, #define downto -->
. If you're feeling evil, you can do #define for while(
and #define do )
(and #define done ;
) and write for x downto 0 do printf("%dn", x) done
Oh, the humanity...– Chris Lutz
Mar 4 '10 at 7:07
#define upto ++<
#define downto -->
#define for while(
#define do )
#define done ;
for x downto 0 do printf("%dn", x) done
Opens the possibility of a whole new expressive way of coding, well worth sacrificing a few compiler warnings for: bool CheckNegative(int x) return x<0 ? true :-( false );
– ttt
May 28 '12 at 11:12
22 Answers
22
-->
is not an operator. It is in fact two separate operators, --
and >
.
-->
--
>
The conditional's code decrements x
, while returning x
's original (not decremented) value, and then compares the original value with 0
using the >
operator.
x
x
0
>
To better understand, the statement could be written as follows:
while( (x--) > 0 )
Then again, it does kind of look like some kind of range operator in that context.
– Charles Salvia
Oct 29 '09 at 7:14
Saying that x is post-decremented and then compared to 0 is the same as saying x is decremented after being compared to 0
– Charles Salvia
Oct 29 '09 at 8:35
In Java it also compiles :)
– Steven Devijver
Feb 12 '13 at 8:07
The name for it, @Jay, is bad programming style :-) This is evidenced by the fact the question was asked in the first place. It makes much more sense to textually bind operators to the thing they're operating on rather than something unrelated, so
while (x-- > 0)
would be more apt. It also makes it more obvious what's going on (at least in a fixed-font editor) meaning that the parentheses in this answer would not be necessary.– paxdiablo
Feb 15 '16 at 1:28
while (x-- > 0)
So to explain the "-->" operator you have to use the "( (" operator and the "--) >" operator?
– Ira Baxter
Apr 2 '16 at 20:37
Or for something completely different... x slides to 0
while (x --
> 0)
printf("%d ", x);
Not so mathematical, but... every picture paints a thousand words. ...
Sorry, I don't get this one. How does this work?
– mafu
Mar 7 '12 at 10:48
@mafutrct - As I remember it in C just appends the next line as if there was not a line break. The s here basically do nothing.
– Hogan
Mar 10 '12 at 4:37
IIRC, K&R C allowed whitespace between the '-'s in the decrement operator, in which case you could have the backslashes in the middle of it, which would look even cooler. :)
– Jules
Jul 13 '13 at 9:23
@ArnavBorborah it's an old expression meaning
why waste words when a picture does a better job
, used as a joke in this context. (there are in fact 2 keywords while
and printf
)– unsynchronized
Sep 10 '16 at 11:36
why waste words when a picture does a better job
while
printf
Ah yes, the obscure slide operator. How could I forget!
– demonkoryu
Oct 27 '17 at 10:00
That's a very complicated operator, so even ISO/IEC JTC1 (Joint Technical Committee 1) placed its description in two different parts of the C++ Standard.
Joking aside, they are two different operators: --
and >
described respectively in §5.2.6/2 and §5.9 of the C++03 Standard.
--
>
It's equivalent to
while (x-- > 0)
While this might help many to understand the code, a few lines to explain it properly would be helpful.
– Dukeling
May 22 '15 at 12:57
@Dukeling
x--
is short hand notation for x-1
– Albert Renshaw
Mar 6 '16 at 5:15
x--
x-1
@AlbertRenshaw I'm sure the year old comment you responded to meant that an explanation of it not being a single operator, but rather two operators that were placed in a confusion fashion would have been helpful.
– mah
Mar 6 '16 at 18:17
@mbomb007 Sorry I meant to say x-=1 —— was up late haha
– Albert Renshaw
Mar 8 '16 at 21:01
@AlbertRenshaw but
x -= 1
and x--
are not the same... If you want to write it like that then it would have to be while (x >= 0) x -= 1;
– Krzysztof Karski
May 16 '16 at 6:27
x -= 1
x--
while (x >= 0) x -= 1;
x
can go to zero even faster in the opposite direction:
x
int x = 10;
while( 0 <---- x )
printf("%d ", x);
8 6 4 2
8 6 4 2
You can control speed with an arrow!
int x = 100;
while( 0 <-------------------- x )
printf("%d ", x);
90 80 70 60 50 40 30 20 10
90 80 70 60 50 40 30 20 10
;)
which operating system, this type of output generated, i am using a ubuntu 12.04 in that i had a error message
– Bhuvanesh
Jan 19 '15 at 12:13
Though it should be obvious, to everyone new to C++ reading this: don't do it. Just use augmented assignment if you have need to increment/decrement by more than one.
– Blimeo
Mar 26 '15 at 2:41
Zero with "lasers". while( 0 > - - - - - -- -- -- -- -- ---------- x ) ... same output.
– Samuel Danielson
Mar 9 '16 at 21:54
@phord are you sure it does not compile? --> coliru.stacked-crooked.com/a/5aa89a65e3a86c98
– doc
Mar 24 '16 at 10:43
@doc It compiles in c++, but not in c.
– phord
Mar 25 '16 at 14:58
It's
#include <stdio.h>
int main(void)
int x = 10;
while( x-- > 0 ) // x goes to 0
printf("%d ", x);
return 0;
Just the space make the things look funny, --
decrements and >
compares.
--
>
The usage of -->
has historical relevance. Decrementing was (and still is in some cases), faster than incrementing on the x86 architecture. Using -->
suggests that x
is going to 0
, and appeals to those with mathematical backgrounds.
-->
-->
x
0
Not exactly true. Decrementing and Incrementing take the same amount of time, the benefit of this is that comparison to zero is very fast compared to comparison versus a variable. This is true for many architectures, not just x86. Anything with a JZ instruction (jump if zero). Poking around you can find many "for" loops that are written backwards to save cycles on the compare. This is particularly fast on x86 as the act of decrementing the variable set the zero flag appropriately, so you could then branch without having to explicitly compare the variable.
– burito
Dec 30 '09 at 5:16
Well, decrementing toward zero means you only have to compare against 0 per loop iteration, while iterating toward n means comparing with n each iteration. The former tends to be easier (and on some architectures, is automatically tested after every data register operation).
– Joey Adams
Apr 12 '10 at 15:07
@burrito Although I don't disagree, loops conditioned on non-zero values generally get predicted near perfectly.
– Duncan
Jan 11 '14 at 9:05
Increment and decrement are equally fast, probably on all platforms (definitely on x86). The difference is in testing the loop end condition. To see if the counter has reached zero is practically free - when you decrement a value, a zero flag is set in processor and to detect the end condition you just need to check that flag whereas when you increment a comparison operation is required before end condition can be detected.
– lego
Feb 18 '15 at 11:14
Of course, all this is moot these days, since modern compilers can vectorize and reverse loops automatically.
– Lambda Fairy
Feb 20 '15 at 3:53
while( x-- > 0 )
is how that's parsed.
Utterly geek, but I will be using this:
#define as ;while
int main(int argc, char* argv)
int n = atoi(argv[1]);
do printf("n is %dn", n) as ( n --> 0);
return 0;
I hope I never come across any of your source code…
– mk12
Aug 15 '12 at 1:44
@Mk12 That's not source code...it's hieroglyphics :-)
– raffian
Sep 7 '12 at 3:27
@SAFX - It would be perfectly hieroglyphics with egyptian brackets
– mouviciel
Nov 14 '12 at 10:00
This doesn't compile. C is not Pascal, where the interior of
do ... while
is a statement list. In C it is a block, so it must be do ... while
.– user207421
Sep 11 '16 at 2:20
do ... while
do ... while
@EJP it does compile. The syntax is
do statement while ( expression ) ;
. Having said that, I hope it is understood I meant the example as a joke.– Escualo
Oct 18 '16 at 17:28
do statement while ( expression ) ;
One book I read (I don't remember correctly which book) stated: Compilers try to parse expressions to the biggest token by using the left right rule.
In this case, the expression:
x-->0
Parses to biggest tokens:
token 1: x
token 2: --
token 3: >
token 4: 0
conclude: x-- > 0
The same rule applies to this expression:
a-----b
After parse:
token 1: a
token 2: --
token 3: --
token 4: -
token 5: b
conclude: (a--)-- - b
I hope this helps to understand the complicated expression ^^
Your second explanation is not correct. The compiler will see
a-----b
and think (a--)-- - b
, which does not compile because a--
does not return an lvalue.– Tim Leaf
May 5 '10 at 15:26
a-----b
(a--)-- - b
a--
Additionally,
x
and --
are two separate tokens.– Roland Illig
Jul 2 '10 at 19:20
x
--
This is known as Maximal munch.
– RJFalconer
Mar 13 '14 at 11:09
@DoctorT: it passes the lexer. only semantic pass is capable of emmiting that error. so his explanation is correct.
– v.oddou
Sep 1 '14 at 3:34
As long as you think
-->
is an operator (which is what's implied by having the question that was asked), this answer isn't helpful at all - you'll think token 2 is -->
, not just --
. If you know that -->
isn't an operator, you probably don't have a problem understanding the code in the question, so, unless you have a completely different question, I'm not really sure how this could be useful.– Dukeling
May 22 '15 at 12:33
-->
-->
--
-->
This is exactly the same as
while (x--)
printf("%d ", x);
for non-negative numbers
@DoctorT you mean "non-negative".
– user142019
Jun 5 '11 at 21:38
Shouldn't this be
for(--x++;--x;++x--)
?– Mateen Ulhaq
Dec 4 '11 at 21:32
for(--x++;--x;++x--)
@DoctorT that's what
unsigned
is for– Cole Johnson
Mar 23 '13 at 18:39
unsigned
@MateenUlhaq, that is wrong according to the standard the expression
--x++
has undefined behaviour according to §1.9.15– WorldSEnder
Jun 19 '15 at 2:02
--x++
Anyway, we have a "goes to" operator now. "-->"
is easy to be remembered as a direction, and "while x goes to zero" is meaning-straight.
"-->"
Furthermore, it is a little more efficient than "for (x = 10; x > 0; x --)"
on some platforms.
"for (x = 10; x > 0; x --)"
Goes to cant be true always especially when value of x is negative.
– Ganesh Gopalasubramanian
Nov 13 '09 at 3:22
The other version does not do the same thing - with
for (size_t x=10; x-->0; )
the body of the loop is executed with 9,8,..,0 whereas the other version has 10,9,..,1. It's quite tricky to exit a loop down to zero with an unsigned variable otherwise.– Pete Kirkham
Jun 21 '10 at 8:57
for (size_t x=10; x-->0; )
I think this is a little bit misleading... We don't have a literally "goes to" operator, since we need another
++>
to do the incremental work.– tslmy
Jun 15 '13 at 2:49
++>
@Josh: actually, overflow gives undefined behavior for
int
, so it could just as easily eat your dog as take x
to zero if it starts out negative.– SamB
Dec 6 '13 at 6:57
int
x
This is a very important idiom to me for the reason given in the comnmet by @PeteKirkham, as I often need to do decreasing loops over unsigned quantities all the way to
0
. (For comparison, the idiom of omitting tests for zero, such as writing while (n--)
instead for unsigned n
, buys you nothing and for me greatly hampers readability.) It also has the pleasant property that you specify one more than the initial index, which is usually what you want (e.g., for a loop over an array you specify its size). I also like -->
without space, as this makes the idiom easy to recognise.– Marc van Leeuwen
Aug 30 '14 at 20:08
0
while (n--)
n
-->
This code first compares x and 0 and then decrements x. (Also said in the first answer: You're post-decrementing x and then comparing x and 0 with the >
operator.) See the output of this code:
>
9 8 7 6 5 4 3 2 1 0
We now first compare and then decrement by seeing 0 in the output.
If we want to first decrement and then compare, use this code:
#include <stdio.h>
int main(void)
int x = 10;
while( --x> 0 ) // x goes to 0
printf("%d ", x);
return 0;
That output is:
9 8 7 6 5 4 3 2 1
My compiler will print out 9876543210 when I run this code.
#include <iostream>
int main()
int x = 10;
while( x --> 0 ) // x goes to 0
std::cout << x;
As expected. The while( x-- > 0 )
actually means while( x > 0)
. The x--
post decrements x
.
while( x-- > 0 )
while( x > 0)
x--
x
while( x > 0 )
x--;
std::cout << x;
is a different way of writing the same thing.
It is nice that the original looks like "while x goes to 0" though.
The result is only undefined when you're incrementing/decrementing the same variable more than once in the same statement. It doesn't apply to this situation.
– Tim Leaf
May 5 '10 at 15:30
while( x-- > 0 ) actually means while( x > 0)
- I'm not sure what you were trying to say there, but the way you phrased it implies the --
has no meaning whatsoever, which is obviously very wrong.– Dukeling
May 22 '15 at 12:28
while( x-- > 0 ) actually means while( x > 0)
--
There is a space missing between --
and >
. x
is post decremented, that is, decremented after checking the condition x>0 ?
.
--
>
x
x>0 ?
The space is not missing - C(++) ignores whitespace.
– user529758
Aug 2 '12 at 19:16
@H2CO3 This isn't true in general. There are places where white space must be used to separate tokens, e.g. in
#define foo()
versus #define foo ()
.– Jens
Apr 25 '13 at 21:16
#define foo()
#define foo ()
@Jens How about: "The space is not missing - C(++) ignores unnecessary white space."?
– Kevin P. Rice
Dec 4 '13 at 20:35
--
is the decrement operator and >
is the greater-than operator.
--
>
The two operators are applied as a single one like -->
.
-->
They're applied as the 2 separate operators they are. They're only written misleadingly to look like "a single one".
– underscore_d
Nov 12 '16 at 17:56
It's a combination of two operators. First --
is for decrementing the value, and >
is for checking whether the value is greater than the right-hand operand.
--
>
#include<stdio.h>
int main()
int x = 10;
while (x-- > 0)
printf("%d ",x);
return 0;
The output will be:
9 8 7 6 5 4 3 2 1 0
Actually, x
is post-decrementing and with that condition is being checked. It's not -->
, it's (x--) > 0
x
-->
(x--) > 0
Note: value of x
is changed after the condition is checked, because it post-decrementing. Some similar cases can also occur, for example:
x
--> x-->0
++> x++>0
-->= x-->=0
++>= x++>=0
Except that ++> can hardly be used in a while(). A "goes up to..." operator would be ++<, which doesn't look anywhere as nice. The operator --> is a happy coincidence.
– Florian F
Sep 1 '14 at 9:46
Could
while (0 <-- x)
also work, then?– Ben Leggiero
Jun 15 '15 at 14:00
while (0 <-- x)
@BenLeggiero That could 'work' in the sense of generating code that does something (while infuriating readers who don't like faux-clever code), but the semantics are different, as its use of predecrement means it will execute one fewer iteration. As a contrived example, it would never execute the loop body if
x
started at 1, but while ( (x--) > 0 )
would. edit Eric Lippert covered both in his C# 4 release notes: blogs.msdn.microsoft.com/ericlippert/2010/04/01/…– underscore_d
Nov 12 '16 at 17:57
x
while ( (x--) > 0 )
C and C++ obey the "maximum munch" rule. The same way a---b is translated to (a--) - b
, in your case x-->0
translates to (x--)>0
.
(a--) - b
x-->0
(x--)>0
What the rule says essentially is that going left to right, expressions are formed by taking the maximum of characters which will form an valid expression.
Which is what the OP assumed: that "((a)-->)" was the maximal munch. It turns out that the OP's original assumption was incorrect: "-->" is not a maximum valid operator.
– david
Aug 28 '14 at 0:41
Also known as greedy parsing, if I recall correctly.
– Roy Tinker
Jul 11 '15 at 1:04
@RoyTinker Greedy scanning. The parser has nothing to do with this.
– user207421
Sep 11 '16 at 2:21
Why all the complication?
The simple answer to the original question is just :
#include <stdio.h>
int main()
int x = 10;
while (x > 0)
printf("%d ", x);
x = x-1;
Does the same thing. Not saying you should do it like this, but it does the same thing and would have answered the question in one post.
The x--
is just shorthand for the above, and >
is just a normal greater-than operator
. No big mystery!
x--
>
operator
There's too much people making simple things complicated nowadays ;)
This question is not about complications, but about ** Hidden Features and Dark Corners of C++/STL**
– pix
Oct 27 '16 at 15:32
The program here gives different output than original because x here is decremented after printf. That demonstrates well how "simple answers" are usually Incorrect.
– Öö Tiib
May 13 '17 at 9:30
The OP's way: 9 8 7 6 5 4 3 2 1 0
and The Garry_G way: 10 9 8 7 6 5 4 3 2 1
– Anthony
Dec 15 '17 at 18:33
The OP's way: 9 8 7 6 5 4 3 2 1 0
The Garry_G way: 10 9 8 7 6 5 4 3 2 1
Conventional way we define condition in while loop parenthesis"()
" and terminating condition inside the braces"", but this
--
& >
is a way one defines all at once.
For e.g:
()
--
>
int abc()
int a = 5
while((a--) > 0) // Decrement and comparison both at once
// Code
It says, decrement a
and run the loop till the time a
is greater than 0
a
a
0
Other way it should have been like:
int abc()
int a = 5
while(a > 0)
// Code
a = a -1 // Decrement inside loop
both ways, we do the same thing and achieve the same goals.
This is incorrect. The code in the question does: 'test-write-execute' (test first, write new value, execute the loop), your example is 'test-execute-write'.
– v010dya
Jul 14 '17 at 19:07
If you were to write the function to do the reverse, the syntax is much more obvious needless to say I don't think I had seen it before either:
#include <stdio.h>
int main()
int x 10; while (x ++< 20) // x increments to 20
printf("%d ", x);
Result:
11 12 13 14 15 16 17 18 19 20
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
Or even just proper spacing... I don't think I've ever seen a space between the variable and either
++
or--
before...– Matthew Scharley
Oct 29 '09 at 7:09